Welcome to Simli
The API devs use to add faces to realtime AI agents
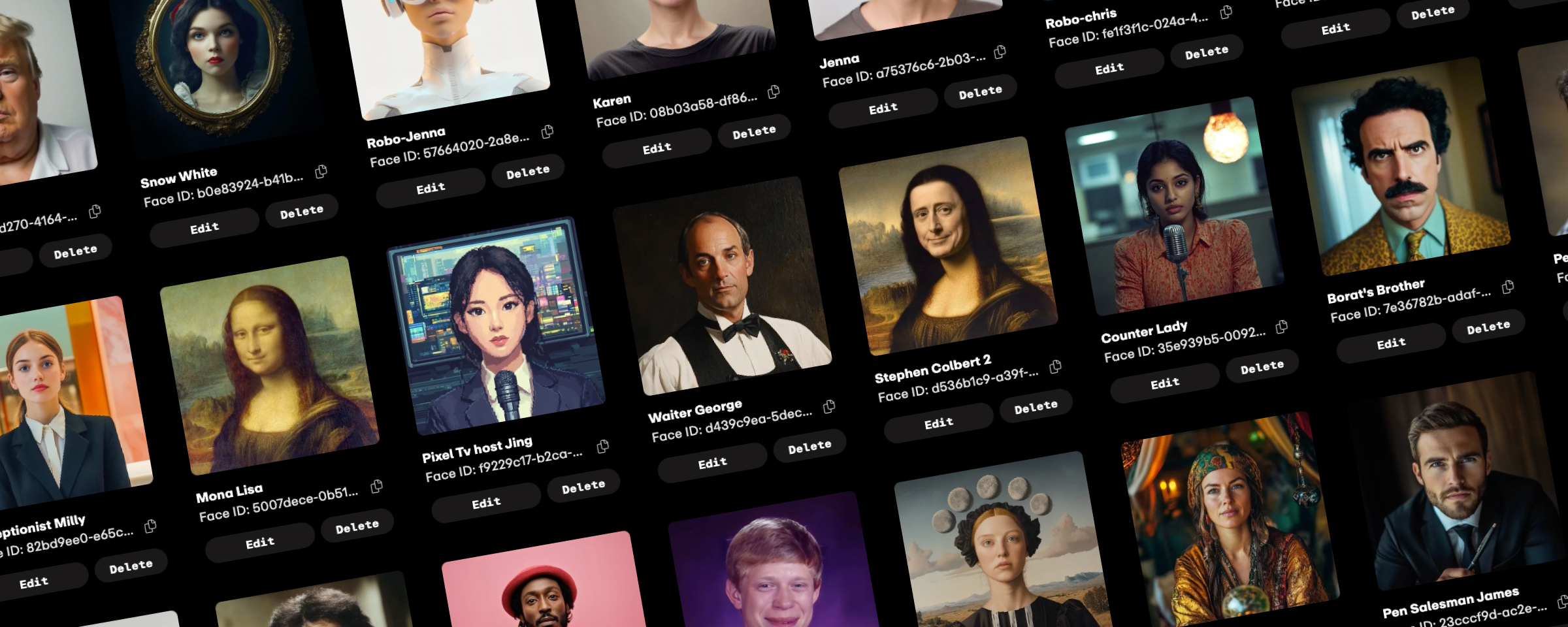
The Simli speech-to-video API lets developers create lip-synced AI avatar interactions. With visual AI avatars, you can create anything! Mock interviews, sales assistants, language learning, coaching, customer-service training, historical characters, and so much more. To see it in action first, try our demo. Ready to make your first one?
Use Simli Widget
Embed a Simli Agent on your website in a few minutes.
Use Simli Auto
Recommended if you want to develop a custom interface for your Simli Agent.
Use an SDK
Recommended if you’re adding a Simli Face to your own custom Agent stack.
Join our community
Share your projects, get technical help, and stay up-to-date with the latest features.